Preview Data
What is preview data?
Preview data encompasses:
- Entities that exist in draft form and have not been published
- Unpublished changes to entities that have been previously published
Currently, Nacelle supports preview data for content from Contentful and Sanity.
Prerequisites
If you use Contentful as a CMS, please ensure that your Contentful Preview Token has been added to your Contentful Connector settings.
If you use Sanity as a CMS, please ensure that your Sanity API Token has been added to your Sanity Connector settings.
Generating a Preview Token
In order to request preview data from either the Storefront API or the Storefront SDK, a Nacelle Preview Token is required. Follow these steps to generate a preview token:
From your space in the Nacelle Dashboard, select Space Settings from the primary navigation pane and then select the API Details tab
In the Storefront API section, click the Create New Key button under the Preview Token field. Then, follow the prompts.
A prompt will now be presented with the newly generated preview token. Copy this key down, as it will not be displayed again. Please take care not to expose the Nacelle Preview Token in your frontend project's production environment variables.
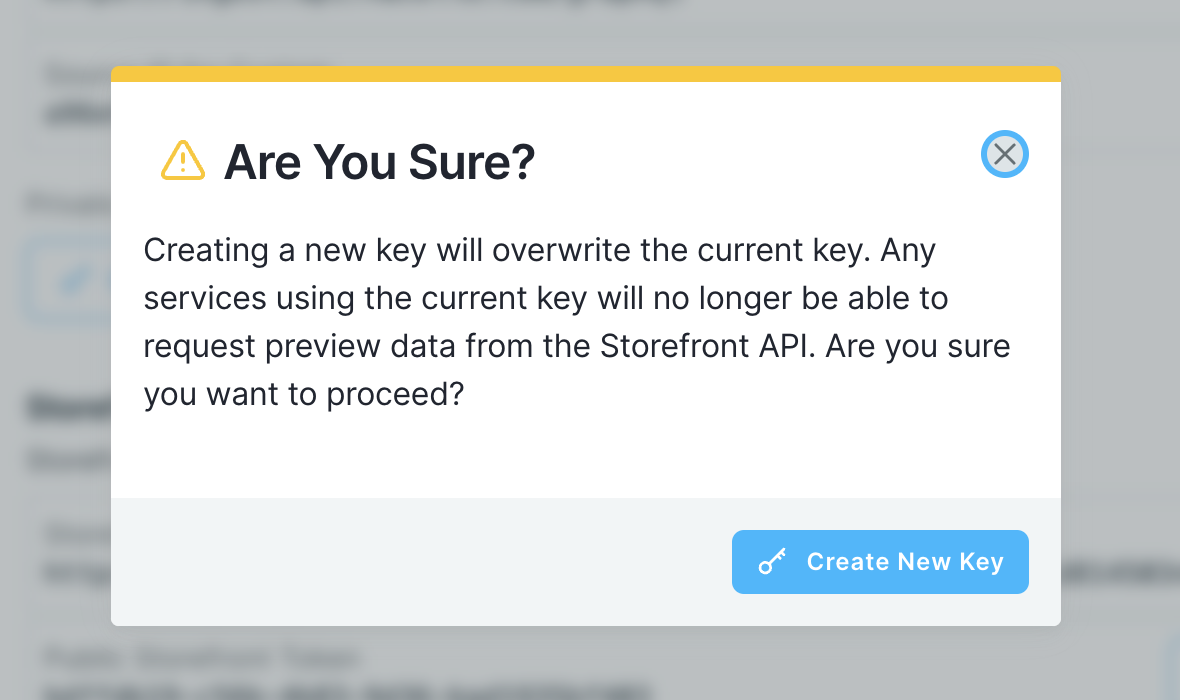
Storefront API
Requests for preview data via the Storefront API will use the standard Storefront API URL with the addition of a preview=true
query parameter.
The
preview
query parameter makes it easy to toggle preview mode in a desktop GraphQL client, without disabling any headers
The Nacelle Preview Token must be supplied via the x-nacelle-preview-token
header. Storefront API GraphQL queries will work when requesting preview data and require no changes.
Example URL:
https://storefront.api.nacelle.com/graphql/v1/spaces/your-space-id?preview=true
Example Headers:
Content-Type: application/json
x-nacelle-preview-token: <your-preview-token>
Storefront SDK
Entering Preview Mode on-the-fly
It's increasingly common for component metaframeworks to offer a Preview Mode that can be entered and exited. This technique eliminates the need for a separate preview site. When working within this type of "on-the-fly" Preview Mode setup, we use the Storefront SDK's setConfig
method to supply a previewToken
.
First, initialize the client normally:
import Storefront from '@nacelle/storefront-sdk';
const client = Storefront({
storefrontEndpoint: '<your-nacelle-storefront-endpoint>',
token: '<your-nacelle-public-storefront-token>'
});
Then use setConfig
to provide the preview token:
client.setConfig({ previewToken: '<your-nacelle-preview-token>' });
Now, client
methods such as content
will return preview data.
Exiting Preview Mode on-the-fly
To exit preview mode, simply pass previewToken: null
to the setConfig
method:
client.setConfig({ previewToken: null });
Initializing the Storefront SDK in Preview Mode
For projects that don't enter Preview Mode on-the-fly and instead rely on a dedicated preview site, you can enable global previews by supplying previewToken
in the Storefront SDK initialization parameters:
import Storefront from '@nacelle/storefront-sdk';
const client = Storefront({
storefrontEndpoint: '<your-nacelle-storefront-endpoint>',
token: '<your-nacelle-public-storefront-token>',
previewToken: '<your-nacelle-preview-token>'
});
Updated about 2 years ago